System.Net Tracing
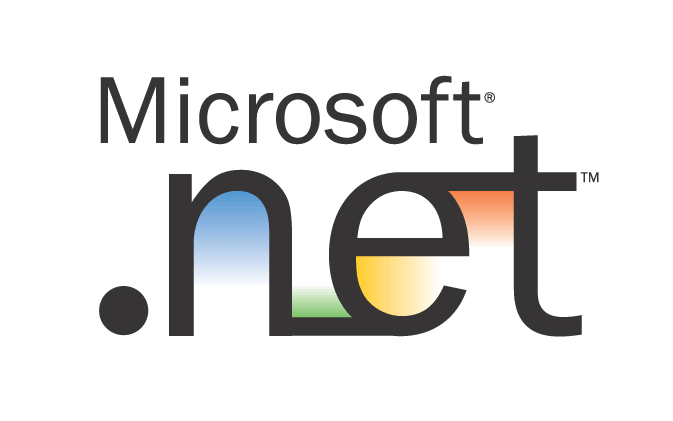
I recently had to troubleshoot a network communication. My app was unable to call an external service endpoint. Troubleshooting network issues can sometimes be as fun as pulling out your own teeth.
In this post I wanted to share a nifty way to perform low-level troubleshooting, which ultimately saved my bacon.
The .NET framework provides tracing capabilities for the System.Net namespace. This is really handy to figure out what is happening when things aren’t working quite as they should.
How Do I Enable It?
Enabling it is just a matter of adding some extra configuration to your app. The System.Net subsystem will then start logging all events to a flat file. Nice and simple.
Just add the below configuration to your application.
<system.diagnostics>
<sources>
<source name="System.Net" tracemode="includehex" maxdatasize="1024">
<listeners>
<add name="System.Net"/>
</listeners>
</source>
<source name="System.Net.Cache">
<listeners>
<add name="System.Net"/>
</listeners>
</source>
<source name="System.Net.Http">
<listeners>
<add name="System.Net"/>
</listeners>
</source>
<source name="System.Net.Sockets">
<listeners>
<add name="System.Net"/>
</listeners>
</source>
<source name="System.Net.WebSockets">
<listeners>
<add name="System.Net"/>
</listeners>
</source>
</sources>
<switches>
<add name="System.Net" value="Verbose"/>
<add name="System.Net.Cache" value="Verbose"/>
<add name="System.Net.Http" value="Verbose"/>
<add name="System.Net.Sockets" value="Verbose"/>
<add name="System.Net.WebSockets" value="Verbose"/>
</switches>
<sharedListeners>
<add name="System.Net"
type="System.Diagnostics.TextWriterTraceListener"
initializeData="C:\\temp\\network.log"
/>
</sharedListeners>
<trace autoflush="true"/>
</system.diagnostics>
For more information, please refer to Microsoft documentation.
https://docs.microsoft.com/en-us/dotnet/framework/network-programming/how-to-configure-network-tracing
Quick Example
To illustrate how it works. Here’s a quick example.
The following code will make a HTTP web request to a website with an “invalid” SSL certificate (a self-signed certificate).
static void Main(string[] args)
{
//
// Make request to site with invalid SSL cert
//
using (var client = new HttpClient())
{
var result = client.GetAsync("https://self-signed.badssl.com/").Result;
}
}
Next, enable network tracing as per above. Then re-run the app. A log file will be generated with detailed low-level network information.
System.Net.Http Verbose: 0 : [2728] HttpClientHandler#45004109::.ctor()
System.Net.Http Verbose: 0 : [2728] Exiting HttpClientHandler#45004109::.ctor()
System.Net Information: 0 : [2728] Current OS installation type is 'Client'.
System.Net.Http Verbose: 0 : [2728] HttpClient#2383799::.ctor(HttpClientHandler#45004109)
System.Net.Http Information: 0 : [2728] Associating HttpClient#2383799 with HttpClientHandler#45004109
System.Net.Http Verbose: 0 : [2728] Exiting HttpClient#2383799::.ctor()
System.Net.Http Verbose: 0 : [2728] HttpClient#2383799::.ctor(HttpClientHandler#45004109)
System.Net.Http Verbose: 0 : [2728] Exiting HttpClient#2383799::.ctor()
System.Net.Http Verbose: 0 : [2728] HttpRequestMessage#21454193::.ctor(Method: GET, Uri: 'https://self-signed.badssl.com/')
System.Net.Http Verbose: 0 : [2728] Exiting HttpRequestMessage#21454193::.ctor()
System.Net.Http Verbose: 0 : [2728] HttpClient#2383799::SendAsync(HttpRequestMessage#21454193: Method: GET, RequestUri: 'https://self-signed.badssl.com/', Version: 1.1, Content: <null>, Headers:
{
})
System.Net.Http Verbose: 0 : [2728] HttpClientHandler#45004109::SendAsync(HttpRequestMessage#21454193)
System.Net Verbose: 0 : [2728] HttpWebRequest#58870012::HttpWebRequest(https://self-signed.badssl.com/#-535670183)
System.Net Information: 0 : [2728] RAS supported: True
System.Net Verbose: 0 : [2728] Exiting HttpWebRequest#58870012::HttpWebRequest()
System.Net Verbose: 0 : [2728] HttpWebRequest#58870012::HttpWebRequest(uri: 'https://self-signed.badssl.com/', connectionGroupName: '45004109')
System.Net Verbose: 0 : [2728] Exiting HttpWebRequest#58870012::HttpWebRequest()
System.Net.Http Information: 0 : [2728] Associating HttpRequestMessage#21454193 with HttpWebRequest#58870012
System.Net Error: 0 : [2728] Can't retrieve proxy settings for Uri 'https://self-signed.badssl.com/'. Error code: 12180.
System.Net Verbose: 0 : [2728] ServicePoint#3741682::ServicePoint(self-signed.badssl.com:443)
System.Net Information: 0 : [2728] Associating HttpWebRequest#58870012 with ServicePoint#3741682
System.Net.Http Verbose: 0 : [2728] Exiting HttpClientHandler#45004109::SendAsync() -> Task`1#54444047
System.Net Verbose: 0 : [0168] HttpWebRequest#58870012::BeginGetResponse()
System.Net.Http Verbose: 0 : [2728] Exiting HttpClient#2383799::SendAsync() -> Task`1#54444047
System.Net Information: 0 : [0168] Associating Connection#4032828 with HttpWebRequest#58870012
System.Net.Sockets Verbose: 0 : [0168] Socket#33711845::Socket(AddressFamily#2)
System.Net.Sockets Verbose: 0 : [0168] Exiting Socket#33711845::Socket()
System.Net.Sockets Verbose: 0 : [0168] Socket#37489757::Socket(AddressFamily#23)
System.Net.Sockets Verbose: 0 : [0168] Exiting Socket#37489757::Socket()
System.Net.Sockets Verbose: 0 : [0168] DNS::TryInternalResolve(self-signed.badssl.com)
System.Net.Sockets Verbose: 0 : [0168] Socket#33711845::BeginConnectEx()
System.Net.Sockets Verbose: 0 : [0168] Socket#33711845::InternalBind(0.0.0.0:0#0)
System.Net.Sockets Verbose: 0 : [0168] Exiting Socket#33711845::InternalBind()
System.Net.Sockets Verbose: 0 : [0168] Exiting Socket#33711845::BeginConnectEx() -> ConnectOverlappedAsyncResult#64828693
System.Net Verbose: 0 : [0168] Exiting HttpWebRequest#58870012::BeginGetResponse() -> ContextAwareResult#10104599
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::EndConnect(ConnectOverlappedAsyncResult#64828693)
System.Net.Sockets Information: 0 : [8708] Socket#33711845 - Created connection from 10.1.0.4:50444 to 104.154.89.105:443.
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::EndConnect()
System.Net.Sockets Verbose: 0 : [8708] Socket#37489757::Close()
System.Net.Sockets Verbose: 0 : [8708] Socket#37489757::Dispose()
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#37489757::Close()
System.Net Information: 0 : [8708] Connection#4032828 - Created connection from 10.1.0.4:50444 to 104.154.89.105:443.
System.Net Information: 0 : [8708] TlsStream#35320229::.ctor(host=self-signed.badssl.com, #certs=0)
System.Net Information: 0 : [8708] Associating HttpWebRequest#58870012 with ConnectStream#17653682
System.Net Information: 0 : [8708] HttpWebRequest#58870012 - Request: GET / HTTP/1.1
System.Net Information: 0 : [8708] ConnectStream#17653682 - Sending headers
{
Host: self-signed.badssl.com
Connection: Keep-Alive
}.
System.Net Information: 0 : [8708] SecureChannel#42194754::.ctor(hostname=self-signed.badssl.com, #clientCertificates=0, encryptionPolicy=RequireEncryption)
System.Net Information: 0 : [8708] Enumerating security packages:
System.Net Information: 0 : [8708] Negotiate
System.Net Information: 0 : [8708] NegoExtender
System.Net Information: 0 : [8708] Kerberos
System.Net Information: 0 : [8708] NTLM
System.Net Information: 0 : [8708] TSSSP
System.Net Information: 0 : [8708] pku2u
System.Net Information: 0 : [8708] WDigest
System.Net Information: 0 : [8708] Schannel
System.Net Information: 0 : [8708] Microsoft Unified Security Protocol Provider
System.Net Information: 0 : [8708] Default TLS SSP
System.Net Information: 0 : [8708] CREDSSP
System.Net Information: 0 : [8708] SecureChannel#42194754 - Left with 0 client certificates to choose from.
System.Net Information: 0 : [8708] AcquireCredentialsHandle(package = Microsoft Unified Security Protocol Provider, intent = Outbound, scc = System.Net.SecureCredential)
System.Net Information: 0 : [8708] InitializeSecurityContext(credential = System.Net.SafeFreeCredential_SECURITY, context = (null), targetName = self-signed.badssl.com, inFlags = ReplayDetect, SequenceDetect, Confidentiality, AllocateMemory, InitManualCredValidation)
System.Net Information: 0 : [8708] InitializeSecurityContext(In-Buffer length=0, Out-Buffer length=178, returned code=ContinueNeeded).
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::BeginSend()
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::BeginSend() -> OverlappedAsyncResult#15688314
System.Net.Sockets Verbose: 0 : [8708] Data from Socket#33711845::PostCompletion
System.Net.Sockets Verbose: 0 : [8708] 00000000 : 16 03 03 00 AD 01 00 00-A9 03 03 5A A8 CE D5 80 : ...........Z....
System.Net.Sockets Verbose: 0 : [8708] 00000010 : B1 F4 DE 9C C8 10 1D D7-65 50 5A 84 79 29 FE CD : ........ePZ.y)..
System.Net.Sockets Verbose: 0 : [8708] 00000020 : B6 86 81 22 F2 6C A0 F6-86 D4 B9 00 00 2A C0 2C : ...".l.......*.,
System.Net.Sockets Verbose: 0 : [8708] 00000030 : C0 2B C0 30 C0 2F 00 9F-00 9E C0 24 C0 23 C0 28 : .+.0./.....$.#.(
System.Net.Sockets Verbose: 0 : [8708] 00000040 : C0 27 C0 0A C0 09 C0 14-C0 13 00 9D 00 9C 00 3D : .'.............=
System.Net.Sockets Verbose: 0 : [8708] 00000050 : 00 3C 00 35 00 2F 00 0A-01 00 00 56 00 00 00 1B : .<.5./.....V....
System.Net.Sockets Verbose: 0 : [8708] 00000060 : 00 19 00 00 16 73 65 6C-66 2D 73 69 67 6E 65 64 : .....self-signed
System.Net.Sockets Verbose: 0 : [8708] 00000070 : 2E 62 61 64 73 73 6C 2E-63 6F 6D 00 0A 00 08 00 : .badssl.com.....
System.Net.Sockets Verbose: 0 : [8708] 00000080 : 06 00 1D 00 17 00 18 00-0B 00 02 01 00 00 0D 00 : ................
System.Net.Sockets Verbose: 0 : [8708] 00000090 : 14 00 12 04 01 05 01 02-01 04 03 05 03 02 03 02 : ................
System.Net.Sockets Verbose: 0 : [8708] 000000A0 : 02 06 01 06 03 00 23 00-00 00 17 00 00 FF 01 00 : ......#.........
System.Net.Sockets Verbose: 0 : [8708] 000000B0 : 01 00 : ..
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::EndSend(OverlappedAsyncResult#15688314)
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::EndSend() -> Int32#178
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::BeginReceive()
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::BeginReceive() -> OverlappedAsyncResult#52307948
System.Net.Sockets Verbose: 0 : [8708] Data from Socket#33711845::PostCompletion
System.Net.Sockets Verbose: 0 : [8708] 00000000 : 16 03 03 00 41 : ....A
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::EndReceive(OverlappedAsyncResult#52307948)
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::EndReceive() -> Int32#5
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::BeginReceive()
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::BeginReceive() -> OverlappedAsyncResult#40535505
System.Net.Sockets Verbose: 0 : [8708] Data from Socket#33711845::PostCompletion
System.Net.Sockets Verbose: 0 : [8708] 00000000 : 02 00 00 3D 03 03 98 F4-BC 59 C7 98 4E 14 E9 AD : ...=.....Y..N...
System.Net.Sockets Verbose: 0 : [8708] 00000010 : 17 83 75 40 DB F4 29 CB-87 A7 4E 1E 55 54 95 7A : ..u@..)...N.UT.z
System.Net.Sockets Verbose: 0 : [8708] 00000020 : F2 E0 C6 7A CD 8A 00 C0-2F 00 00 15 00 00 00 00 : ...z..../.......
System.Net.Sockets Verbose: 0 : [8708] 00000030 : FF 01 00 01 00 00 0B 00-04 03 00 01 02 00 23 00 : ..............#.
System.Net.Sockets Verbose: 0 : [8708] 00000040 : 00 : .
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::EndReceive(OverlappedAsyncResult#40535505)
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::EndReceive() -> Int32#65
System.Net Information: 0 : [8708] InitializeSecurityContext(credential = System.Net.SafeFreeCredential_SECURITY, context = 2ff5b78:2ff7d10, targetName = self-signed.badssl.com, inFlags = ReplayDetect, SequenceDetect, Confidentiality, AllocateMemory, InitManualCredValidation)
System.Net Information: 0 : [8708] InitializeSecurityContext(In-Buffers count=2, Out-Buffer length=0, returned code=ContinueNeeded).
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::BeginReceive()
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::BeginReceive() -> OverlappedAsyncResult#34678979
System.Net.Sockets Verbose: 0 : [8708] Data from Socket#33711845::PostCompletion
System.Net.Sockets Verbose: 0 : [8708] 00000000 : 16 03 03 03 87 : .....
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::EndReceive(OverlappedAsyncResult#34678979)
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::EndReceive() -> Int32#5
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::BeginReceive()
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::BeginReceive() -> OverlappedAsyncResult#66166301
System.Net.Sockets Verbose: 0 : [8708] Data from Socket#33711845::PostCompletion
System.Net.Sockets Verbose: 0 : [8708] 00000000 : 0B 00 03 83 00 03 80 00-03 7D 30 82 03 79 30 82 : .........}0..y0.
System.Net.Sockets Verbose: 0 : [8708] 00000010 : 02 61 A0 03 02 01 02 02-09 00 86 FB 4D C8 E5 DD : .a..........M...
System.Net.Sockets Verbose: 0 : [8708] 00000020 : 0F 18 30 0D 06 09 2A 86-48 86 F7 0D 01 01 0B 05 : ..0...*.H.......
System.Net.Sockets Verbose: 0 : [8708] 00000030 : 00 30 62 31 0B 30 09 06-03 55 04 06 13 02 55 53 : .0b1.0...U....US
System.Net.Sockets Verbose: 0 : [8708] 00000040 : 31 13 30 11 06 03 55 04-08 0C 0A 43 61 6C 69 66 : 1.0...U....Calif
System.Net.Sockets Verbose: 0 : [8708] 00000050 : 6F 72 6E 69 61 31 16 30-14 06 03 55 04 07 0C 0D : ornia1.0...U....
System.Net.Sockets Verbose: 0 : [8708] 00000060 : 53 61 6E 20 46 72 61 6E-63 69 73 63 6F 31 0F 30 : San Francisco1.0
System.Net.Sockets Verbose: 0 : [8708] 00000070 : 0D 06 03 55 04 0A 0C 06-42 61 64 53 53 4C 31 15 : ...U....BadSSL1.
System.Net.Sockets Verbose: 0 : [8708] 00000080 : 30 13 06 03 55 04 03 0C-0C 2A 2E 62 61 64 73 73 : 0...U....*.badss
System.Net.Sockets Verbose: 0 : [8708] 00000090 : 6C 2E 63 6F 6D 30 1E 17-0D 31 36 30 38 30 38 32 : l.com0...1608082
System.Net.Sockets Verbose: 0 : [8708] 000000A0 : 31 31 37 30 35 5A 17 0D-31 38 30 38 30 38 32 31 : 11705Z..18080821
System.Net.Sockets Verbose: 0 : [8708] 000000B0 : 31 37 30 35 5A 30 62 31-0B 30 09 06 03 55 04 06 : 1705Z0b1.0...U..
System.Net.Sockets Verbose: 0 : [8708] 000000C0 : 13 02 55 53 31 13 30 11-06 03 55 04 08 0C 0A 43 : ..US1.0...U....C
System.Net.Sockets Verbose: 0 : [8708] 000000D0 : 61 6C 69 66 6F 72 6E 69-61 31 16 30 14 06 03 55 : alifornia1.0...U
System.Net.Sockets Verbose: 0 : [8708] 000000E0 : 04 07 0C 0D 53 61 6E 20-46 72 61 6E 63 69 73 63 : ....San Francisc
System.Net.Sockets Verbose: 0 : [8708] 000000F0 : 6F 31 0F 30 0D 06 03 55-04 0A 0C 06 42 61 64 53 : o1.0...U....BadS
System.Net.Sockets Verbose: 0 : [8708] 00000100 : 53 4C 31 15 30 13 06 03-55 04 03 0C 0C 2A 2E 62 : SL1.0...U....*.b
System.Net.Sockets Verbose: 0 : [8708] 00000110 : 61 64 73 73 6C 2E 63 6F-6D 30 82 01 22 30 0D 06 : adssl.com0.."0..
System.Net.Sockets Verbose: 0 : [8708] 00000120 : 09 2A 86 48 86 F7 0D 01-01 01 05 00 03 82 01 0F : .*.H............
System.Net.Sockets Verbose: 0 : [8708] 00000130 : 00 30 82 01 0A 02 82 01-01 00 C2 04 EC F8 8C EE : .0..............
System.Net.Sockets Verbose: 0 : [8708] 00000140 : 04 C2 B3 D8 50 D5 70 58-CC 93 18 EB 5C A8 68 49 : ....P.pX....\.hI
System.Net.Sockets Verbose: 0 : [8708] 00000150 : B0 22 B5 F9 95 9E B1 2B-2C 76 3E 6C C0 4B 60 4C : .".....+,v>l.K`L
System.Net.Sockets Verbose: 0 : [8708] 00000160 : 4C EA B2 B4 C0 0F 80 B6-B0 F9 72 C9 86 02 F9 5C : L.........r....\
System.Net.Sockets Verbose: 0 : [8708] 00000170 : 41 5D 13 2B 7F 71 C4 4B-BC E9 94 2E 50 37 A6 67 : A].+.q.K....P7.g
System.Net.Sockets Verbose: 0 : [8708] 00000180 : 1C 61 8C F6 41 42 C5 46-D3 16 87 27 9F 74 EB 0A : .a..AB.F...'.t..
System.Net.Sockets Verbose: 0 : [8708] 00000190 : 9D 11 52 26 21 73 6C 84-4C 79 55 E4 D1 6B E8 06 : ..R&!sl.LyU..k..
System.Net.Sockets Verbose: 0 : [8708] 000001A0 : 3D 48 15 52 AD B3 28 DB-AA FF 6E FF 60 95 4A 77 : =H.R..(...n.`.Jw
System.Net.Sockets Verbose: 0 : [8708] 000001B0 : 6B 39 F1 24 D1 31 B6 DD-4D C0 C4 FC 53 B9 6D 42 : k9.$.1..M...S.mB
System.Net.Sockets Verbose: 0 : [8708] 000001C0 : AD B5 7C FE AE F5 15 D2-33 48 E7 22 71 C7 C2 14 : ..|.....3H."q...
System.Net.Sockets Verbose: 0 : [8708] 000001D0 : 7A 6C 28 EA 37 4A DF EA-6C B5 72 B4 7E 5A A2 16 : zl(.7J..l.r.~Z..
System.Net.Sockets Verbose: 0 : [8708] 000001E0 : DC 69 B1 57 44 DB 0A 12-AB DE C3 0F 47 74 5C 41 : .i.WD.......Gt\A
System.Net.Sockets Verbose: 0 : [8708] 000001F0 : 22 E1 9A F9 1B 93 E6 AD-22 06 29 2E B1 BA 49 1C : ".......".)...I.
System.Net.Sockets Verbose: 0 : [8708] 00000200 : 0C 27 9E A3 FB 8B F7 40-72 00 AC 92 08 D9 8C 57 : .'[email protected]
System.Net.Sockets Verbose: 0 : [8708] 00000210 : 84 53 81 05 CB E6 FE 6B-54 98 40 27 85 C7 10 BB : .S.....kT.@'....
System.Net.Sockets Verbose: 0 : [8708] 00000220 : 73 70 EF 69 18 41 07 45-55 7C F9 64 3F 3D 2C C3 : sp.i.A.EU|.d?=,.
System.Net.Sockets Verbose: 0 : [8708] 00000230 : A9 7C EB 93 1A 4C 86 D1-CA 85 02 03 01 00 01 A3 : .|...L..........
System.Net.Sockets Verbose: 0 : [8708] 00000240 : 32 30 30 30 09 06 03 55-1D 13 04 02 30 00 30 23 : 2000...U....0.0#
System.Net.Sockets Verbose: 0 : [8708] 00000250 : 06 03 55 1D 11 04 1C 30-1A 82 0C 2A 2E 62 61 64 : ..U....0...*.bad
System.Net.Sockets Verbose: 0 : [8708] 00000260 : 73 73 6C 2E 63 6F 6D 82-0A 62 61 64 73 73 6C 2E : ssl.com..badssl.
System.Net.Sockets Verbose: 0 : [8708] 00000270 : 63 6F 6D 30 0D 06 09 2A-86 48 86 F7 0D 01 01 0B : com0...*.H......
System.Net.Sockets Verbose: 0 : [8708] 00000280 : 05 00 03 82 01 01 00 B5-B8 A5 A7 79 D9 3E D5 37 : ...........y.>.7
System.Net.Sockets Verbose: 0 : [8708] 00000290 : 0D A7 14 C8 CF 1F DF 19-24 D0 10 42 05 B2 AF 71 : ........$..B...q
System.Net.Sockets Verbose: 0 : [8708] 000002A0 : F6 2A 56 4D E8 81 46 B2-89 CE 9C 20 BF 0E 8F 01 : .*VM..F.... ....
System.Net.Sockets Verbose: 0 : [8708] 000002B0 : 3F 1E 81 9C FD CF 17 0A-2F 58 C6 AD D5 5B E7 45 : ?......./X...[.E
System.Net.Sockets Verbose: 0 : [8708] 000002C0 : 1A 02 F2 5C 3F DF 47 84-6B 35 AA 05 11 99 58 FC : ...\?.G.k5....X.
System.Net.Sockets Verbose: 0 : [8708] 000002D0 : FA 2C 74 C4 DC 33 41 25-D7 3C C7 3E A6 87 CA BD : .,t..3A%.<.>....
System.Net.Sockets Verbose: 0 : [8708] 000002E0 : 44 95 FF 3C 08 FE 61 81-8D 12 54 EE EA A8 F8 F5 : D..<..a...T.....
System.Net.Sockets Verbose: 0 : [8708] 000002F0 : 84 F4 04 48 4C 17 85 3E-75 34 34 1B CC B7 C7 85 : ...HL..>u44.....
System.Net.Sockets Verbose: 0 : [8708] 00000300 : 8D 7E 2B BD A4 6A B2 AE-9F 1D 03 37 A2 74 C7 D0 : .~+..j.....7.t..
System.Net.Sockets Verbose: 0 : [8708] 00000310 : FE 95 34 D4 FA 68 3D 30-CB BD 10 CD 92 63 2C 00 : ..4..h=0.....c,.
System.Net.Sockets Verbose: 0 : [8708] 00000320 : 13 44 D5 F5 C1 7C 9C 43-0C 6C 1F AB 5C 58 D6 3D : .D...|.C.l..\X.=
System.Net.Sockets Verbose: 0 : [8708] 00000330 : B7 16 6E 81 A2 5C 19 0B-6C BB 9F 5F 61 FA F3 18 : ..n..\..l.._a...
System.Net.Sockets Verbose: 0 : [8708] 00000340 : 55 66 C6 A2 8B EB 7F 6F-E2 6D 3B ED 71 3C 9F 0E : Uf.....o.m;.q<..
System.Net.Sockets Verbose: 0 : [8708] 00000350 : 8A 7B 82 5E 58 CB 73 A7-71 9C 68 90 58 F6 D6 B5 : .{.^X.s.q.h.X...
System.Net.Sockets Verbose: 0 : [8708] 00000360 : 19 A4 B8 31 F5 E6 B8 6B-DC F7 17 31 33 E6 E7 F2 : ...1...k...13...
System.Net.Sockets Verbose: 0 : [8708] 00000370 : 9E AD 37 46 11 D3 13 57-AE FA 00 F3 30 41 74 18 : ..7F...W....0At.
System.Net.Sockets Verbose: 0 : [8708] 00000380 : D8 46 1A C2 AA 78 0E : .F...x.
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::EndReceive(OverlappedAsyncResult#66166301)
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::EndReceive() -> Int32#903
System.Net Information: 0 : [8708] InitializeSecurityContext(credential = System.Net.SafeFreeCredential_SECURITY, context = 2ff5b78:2ff7d10, targetName = self-signed.badssl.com, inFlags = ReplayDetect, SequenceDetect, Confidentiality, AllocateMemory, InitManualCredValidation)
System.Net Information: 0 : [8708] InitializeSecurityContext(In-Buffers count=2, Out-Buffer length=0, returned code=ContinueNeeded).
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::BeginReceive()
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::BeginReceive() -> OverlappedAsyncResult#39774547
System.Net.Sockets Verbose: 0 : [8708] Data from Socket#33711845::PostCompletion
System.Net.Sockets Verbose: 0 : [8708] 00000000 : 16 03 03 01 4D : ....M
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::EndReceive(OverlappedAsyncResult#39774547)
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::EndReceive() -> Int32#5
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::BeginReceive()
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::BeginReceive() -> OverlappedAsyncResult#12611187
System.Net.Sockets Verbose: 0 : [8708] Data from Socket#33711845::PostCompletion
System.Net.Sockets Verbose: 0 : [8708] 00000000 : 0C 00 01 49 03 00 17 41-04 14 52 9B BE 20 1A C1 : ...I...A..R.. ..
System.Net.Sockets Verbose: 0 : [8708] 00000010 : 39 39 20 FC 48 65 7F FA-B5 4B E2 E2 07 92 E8 AD : 99 .He...K......
System.Net.Sockets Verbose: 0 : [8708] 00000020 : DA F6 AD 06 57 09 81 EE-5D C6 23 22 31 24 58 2F : ....W...].#"1$X/
System.Net.Sockets Verbose: 0 : [8708] 00000030 : D4 1A 5A 73 5C 18 4C 0F-E6 15 72 61 5F B1 91 B8 : ..Zs\.L...ra_...
System.Net.Sockets Verbose: 0 : [8708] 00000040 : 3C 64 47 40 B3 A0 DD DA-FC 06 01 01 00 B8 36 3C : <[email protected]<
System.Net.Sockets Verbose: 0 : [8708] 00000050 : DA C7 06 13 93 C1 1E 11-4C 3B 4C 9F 2E BF 76 60 : ........L;L...v`
System.Net.Sockets Verbose: 0 : [8708] 00000060 : 68 A5 9D 68 72 95 70 E7-86 D0 42 23 13 13 3E 25 : h..hr.p...B#..>%
System.Net.Sockets Verbose: 0 : [8708] 00000070 : 10 D9 2E A2 92 B1 CC 92-C8 AE FE C8 35 28 06 9B : ............5(..
System.Net.Sockets Verbose: 0 : [8708] 00000080 : 75 3B 42 05 8B 41 BA CE-84 86 20 EC B8 50 E1 BF : u;B..A.... ..P..
System.Net.Sockets Verbose: 0 : [8708] 00000090 : D2 6F BB 10 7B DE 5A 53-66 73 C1 32 90 64 0D 20 : .o..{.ZSfs.2.d.
System.Net.Sockets Verbose: 0 : [8708] 000000A0 : B7 28 B7 24 D9 88 CE D9-3C 7C F9 72 B1 39 7C 61 : .(.$....<|.r.9|a
System.Net.Sockets Verbose: 0 : [8708] 000000B0 : D1 94 7B DA DE 5F 2D 22-37 E0 4E 94 83 C9 D6 21 : ..{.._-"7.N....!
System.Net.Sockets Verbose: 0 : [8708] 000000C0 : F6 E8 A7 11 E1 F0 DD 32-42 DE 42 7C 19 02 8A 6C : .......2B.B|...l
System.Net.Sockets Verbose: 0 : [8708] 000000D0 : 1A ED 04 7C D1 3E 48 E1-A3 7E 7F C0 DC 95 65 5E : ...|.>H..~....e^
System.Net.Sockets Verbose: 0 : [8708] 000000E0 : 09 BA CC D1 C4 09 91 1E-C1 57 9F 07 39 5C 8F 00 : .........W..9\..
System.Net.Sockets Verbose: 0 : [8708] 000000F0 : E7 72 EA 9B 79 64 4D C3-C1 14 26 26 16 F9 36 D6 : .r..ydM...&&..6.
System.Net.Sockets Verbose: 0 : [8708] 00000100 : 1B 91 29 B4 70 83 D0 A9-8C 7E 69 48 1D CC 1A 89 : ..).p....~iH....
System.Net.Sockets Verbose: 0 : [8708] 00000110 : EB 41 39 76 9E DE 6D 94-88 74 01 D4 38 68 1B E1 : .A9v..m..t..8h..
System.Net.Sockets Verbose: 0 : [8708] 00000120 : 89 2E DF 24 5A DD 6F FB-56 FD E3 77 46 35 F6 FC : ...$Z.o.V..wF5..
System.Net.Sockets Verbose: 0 : [8708] 00000130 : 2E 17 70 E7 9B F1 9B DC-50 08 7F 4B 64 D3 49 A9 : ..p.....P..Kd.I.
System.Net.Sockets Verbose: 0 : [8708] 00000140 : E4 C8 DF DE 50 BB 24 D0-0B FC A0 86 45 : ....P.$.....E
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::EndReceive(OverlappedAsyncResult#12611187)
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::EndReceive() -> Int32#333
System.Net Information: 0 : [8708] InitializeSecurityContext(credential = System.Net.SafeFreeCredential_SECURITY, context = 2ff5b78:2ff7d10, targetName = self-signed.badssl.com, inFlags = ReplayDetect, SequenceDetect, Confidentiality, AllocateMemory, InitManualCredValidation)
System.Net Information: 0 : [8708] InitializeSecurityContext(In-Buffers count=2, Out-Buffer length=0, returned code=ContinueNeeded).
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::BeginReceive()
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::BeginReceive() -> OverlappedAsyncResult#30180123
System.Net.Sockets Verbose: 0 : [8708] Data from Socket#33711845::PostCompletion
System.Net.Sockets Verbose: 0 : [8708] 00000000 : 16 03 03 00 04 : .....
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::EndReceive(OverlappedAsyncResult#30180123)
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::EndReceive() -> Int32#5
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::BeginReceive()
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::BeginReceive() -> OverlappedAsyncResult#2808346
System.Net.Sockets Verbose: 0 : [8708] Data from Socket#33711845::PostCompletion
System.Net.Sockets Verbose: 0 : [8708] 00000000 : 0E 00 00 00 : ....
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::EndReceive(OverlappedAsyncResult#2808346)
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::EndReceive() -> Int32#4
System.Net Information: 0 : [8708] InitializeSecurityContext(credential = System.Net.SafeFreeCredential_SECURITY, context = 2ff5b78:2ff7d10, targetName = self-signed.badssl.com, inFlags = ReplayDetect, SequenceDetect, Confidentiality, AllocateMemory, InitManualCredValidation)
System.Net Information: 0 : [8708] InitializeSecurityContext(In-Buffers count=2, Out-Buffer length=126, returned code=ContinueNeeded).
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::BeginSend()
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::BeginSend() -> OverlappedAsyncResult#14333193
System.Net.Sockets Verbose: 0 : [8708] Data from Socket#33711845::PostCompletion
System.Net.Sockets Verbose: 0 : [8708] 00000000 : 16 03 03 00 46 10 00 00-42 41 04 2F 0B 94 47 39 : ....F...BA./..G9
System.Net.Sockets Verbose: 0 : [8708] 00000010 : A9 29 0B EC DA CD 03 A2-59 95 72 57 59 7D 60 D4 : .)......Y.rWY}`.
System.Net.Sockets Verbose: 0 : [8708] 00000020 : 08 02 36 AB 5C 5E 79 A7-A7 92 46 01 4D 37 93 C0 : ..6.\^y...F.M7..
System.Net.Sockets Verbose: 0 : [8708] 00000030 : 4C 4F FC 45 EC 0E 7D A9-19 E2 64 DA 99 47 68 14 : LO.E..}...d..Gh.
System.Net.Sockets Verbose: 0 : [8708] 00000040 : 59 F8 4E BE 1F 6A F5 21-EC E0 51 14 03 03 00 01 : Y.N..j.!..Q.....
System.Net.Sockets Verbose: 0 : [8708] 00000050 : 01 16 03 03 00 28 00 00-00 00 00 00 00 00 6C 2B : .....(........l+
System.Net.Sockets Verbose: 0 : [8708] 00000060 : 3C 47 D2 7E C2 93 ED 22-DC 2D DE 7C 6F B2 1B CB : <G.~...".-.|o...
System.Net.Sockets Verbose: 0 : [8708] 00000070 : 3C F9 C0 B2 FF D1 86 7D-2A 05 8F 16 4A D1 : <......}*...J.
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::EndSend(OverlappedAsyncResult#14333193)
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::EndSend() -> Int32#126
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::BeginReceive()
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::BeginReceive() -> OverlappedAsyncResult#13009416
System.Net.Sockets Verbose: 0 : [8708] Data from Socket#33711845::PostCompletion
System.Net.Sockets Verbose: 0 : [8708] 00000000 : 16 03 03 00 DA : .....
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::EndReceive(OverlappedAsyncResult#13009416)
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::EndReceive() -> Int32#5
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::BeginReceive()
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::BeginReceive() -> OverlappedAsyncResult#41728762
System.Net.Sockets Verbose: 0 : [8708] Data from Socket#33711845::PostCompletion
System.Net.Sockets Verbose: 0 : [8708] 00000000 : 04 00 00 D6 00 00 01 2C-00 D0 9A 6C 5E 02 5E 8F : .......,...l^.^.
System.Net.Sockets Verbose: 0 : [8708] 00000010 : 5F E2 EF 71 8E 75 62 B7-F1 F0 D2 D4 FF F4 3E A7 : _..q.ub.......>.
System.Net.Sockets Verbose: 0 : [8708] 00000020 : 66 44 35 F9 A1 D1 17 13-CA 16 A4 1D C6 27 6C 68 : fD5..........'lh
System.Net.Sockets Verbose: 0 : [8708] 00000030 : 0F 88 69 C1 F9 A1 16 86-6D 51 C9 93 57 A2 75 B8 : ..i.....mQ..W.u.
System.Net.Sockets Verbose: 0 : [8708] 00000040 : 93 43 3D 3F 87 75 D1 FD-42 C0 70 09 0E 0C 71 19 : .C=?.u..B.p...q.
System.Net.Sockets Verbose: 0 : [8708] 00000050 : E2 FE 06 D0 BF 07 13 90-2F 91 0C C2 60 97 61 38 : ......../...`.a8
System.Net.Sockets Verbose: 0 : [8708] 00000060 : 77 A8 1A B1 96 A9 7B 67-0B B1 A1 C7 0C 1A F4 57 : w.....{g.......W
System.Net.Sockets Verbose: 0 : [8708] 00000070 : 3A EF D1 0E 35 3D C8 4C-A2 F8 A2 E1 F0 06 1D 4C : :...5=.L.......L
System.Net.Sockets Verbose: 0 : [8708] 00000080 : B8 4A 0B 0E 71 3C 24 CE-9B C1 37 A3 F4 5C 41 0D : .J..q<$...7..\A.
System.Net.Sockets Verbose: 0 : [8708] 00000090 : D0 D1 15 C7 62 1A CE DB-23 B9 06 9B 91 B8 48 B5 : ....b...#.....H.
System.Net.Sockets Verbose: 0 : [8708] 000000A0 : C3 12 85 A0 EC 2A D5 40-13 80 55 F3 17 DD 81 4C : .....*[email protected]
System.Net.Sockets Verbose: 0 : [8708] 000000B0 : C7 2C FD 53 5D 04 8D 23-86 D0 2F B5 19 1D BD A2 : .,.S]..#../.....
System.Net.Sockets Verbose: 0 : [8708] 000000C0 : B6 62 58 0A 0E E9 43 3D-3A AA B0 B5 21 4E 8D A4 : .bX...C=:...!N..
System.Net.Sockets Verbose: 0 : [8708] 000000D0 : CE F3 95 12 34 C2 1A 8E-F1 C6 : ....4.....
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::EndReceive(OverlappedAsyncResult#41728762)
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::EndReceive() -> Int32#218
System.Net Information: 0 : [8708] InitializeSecurityContext(credential = System.Net.SafeFreeCredential_SECURITY, context = 2ff5b78:2ff7d10, targetName = self-signed.badssl.com, inFlags = ReplayDetect, SequenceDetect, Confidentiality, AllocateMemory, InitManualCredValidation)
System.Net Information: 0 : [8708] InitializeSecurityContext(In-Buffers count=2, Out-Buffer length=0, returned code=ContinueNeeded).
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::BeginReceive()
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::BeginReceive() -> OverlappedAsyncResult#2174563
System.Net.Sockets Verbose: 0 : [8708] Data from Socket#33711845::PostCompletion
System.Net.Sockets Verbose: 0 : [8708] 00000000 : 14 03 03 00 01 : .....
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::EndReceive(OverlappedAsyncResult#2174563)
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::EndReceive() -> Int32#5
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::BeginReceive()
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::BeginReceive() -> OverlappedAsyncResult#63062333
System.Net.Sockets Verbose: 0 : [8708] Data from Socket#33711845::PostCompletion
System.Net.Sockets Verbose: 0 : [8708] 00000000 : 01 : .
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::EndReceive(OverlappedAsyncResult#63062333)
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::EndReceive() -> Int32#1
System.Net Information: 0 : [8708] InitializeSecurityContext(credential = System.Net.SafeFreeCredential_SECURITY, context = 2ff5b78:2ff7d10, targetName = self-signed.badssl.com, inFlags = ReplayDetect, SequenceDetect, Confidentiality, AllocateMemory, InitManualCredValidation)
System.Net Information: 0 : [8708] InitializeSecurityContext(In-Buffers count=2, Out-Buffer length=0, returned code=ContinueNeeded).
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::BeginReceive()
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::BeginReceive() -> OverlappedAsyncResult#16868352
System.Net.Sockets Verbose: 0 : [8708] Data from Socket#33711845::PostCompletion
System.Net.Sockets Verbose: 0 : [8708] 00000000 : 16 03 03 00 28 : ....(
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::EndReceive(OverlappedAsyncResult#16868352)
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::EndReceive() -> Int32#5
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::BeginReceive()
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::BeginReceive() -> OverlappedAsyncResult#19420176
System.Net.Sockets Verbose: 0 : [8708] Data from Socket#33711845::PostCompletion
System.Net.Sockets Verbose: 0 : [8708] 00000000 : 6E FD 11 64 39 49 3C DD-63 15 1B 5E 87 5F 27 1A : n..d9I<.c..^._'.
System.Net.Sockets Verbose: 0 : [8708] 00000010 : 8B BC DF 7E 03 9E 0B 44-79 EB AE 74 AE 45 C0 83 : ...~...Dy..t.E..
System.Net.Sockets Verbose: 0 : [8708] 00000020 : 90 6C 2D 05 47 20 81 61- : .l-.G .a
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::EndReceive(OverlappedAsyncResult#19420176)
System.Net.Sockets Verbose: 0 : [8708] Exiting Socket#33711845::EndReceive() -> Int32#40
System.Net Information: 0 : [8708] InitializeSecurityContext(credential = System.Net.SafeFreeCredential_SECURITY, context = 2ff5b78:2ff7d10, targetName = self-signed.badssl.com, inFlags = ReplayDetect, SequenceDetect, Confidentiality, AllocateMemory, InitManualCredValidation)
System.Net Information: 0 : [8708] InitializeSecurityContext(In-Buffers count=2, Out-Buffer length=0, returned code=OK).
System.Net Information: 0 : [8708] Remote certificate: [Version]
V3
[Subject]
CN=*.badssl.com, O=BadSSL, L=San Francisco, S=California, C=US
Simple Name: *.badssl.com
DNS Name: badssl.com
[Issuer]
CN=*.badssl.com, O=BadSSL, L=San Francisco, S=California, C=US
Simple Name: *.badssl.com
DNS Name: *.badssl.com
[Serial Number]
0086FB4DC8E5DD0F18
[Not Before]
8/8/2016 9:17:05 PM
[Not After]
8/8/2018 9:17:05 PM
[Thumbprint]
641450D94A65FAEB3B631028D8E86C95431DB811
[Signature Algorithm]
sha256RSA(1.2.840.113549.1.1.11)
[Public Key]
Algorithm: RSA
Length: 2048
Key Blob: 30 82 01 0a 02 82 01 01 00 c2 04 ec f8 8c ee 04 c2 b3 d8 50 d5 70 58 cc 93 18 eb 5c a8 68 49 b0 22 b5 f9 95 9e b1 2b 2c 76 3e 6c c0 4b 60 4c 4c ea b2 b4 c0 0f 80 b6 b0 f9 72 c9 86 02 f9 5c 41 5d 13 2b 7f 71 c4 4b bc e9 94 2e 50 37 a6 67 1c 61 8c f6 41 42 c5 46 d3 16 87 27 9f 74 eb 0a 9d 11 52 26 21 73 6c 84 4c 79 55 e4 d1 6b e8 06 3d 48 15 52 ad b3 28 db aa ff 6e ff 60 95 4a 77 6b 39 f1 24 d1 31 b6 dd 4d c0 c4 fc 53 b9 6d 42 ad b5 7c ....
System.Net Information: 0 : [8708] SecureChannel#42194754 - Remote certificate has errors:
System.Net Information: 0 : [8708] SecureChannel#42194754 - A certificate chain processed, but terminated in a root certificate which is not trusted by the trust provider.
System.Net Information: 0 : [8708] SecureChannel#42194754 - Remote certificate was verified as invalid by the user.
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::Dispose()
System.Net Error: 0 : [8708] Exception in HttpWebRequest#58870012:: - The underlying connection was closed: Could not establish trust relationship for the SSL/TLS secure channel..
System.Net Verbose: 0 : [8708] HttpWebRequest#58870012::EndGetResponse()
System.Net Error: 0 : [8708] Exception in HttpWebRequest#58870012::EndGetResponse - The underlying connection was closed: Could not establish trust relationship for the SSL/TLS secure channel..
System.Net.Http Error: 0 : [8708] Exception in HttpClientHandler#45004109::SendAsync - The underlying connection was closed: Could not establish trust relationship for the SSL/TLS secure channel..
at System.Net.HttpWebRequest.EndGetResponse(IAsyncResult asyncResult)
at System.Net.Http.HttpClientHandler.GetResponseCallback(IAsyncResult ar)
System.Net.Http Error: 0 : [8708] HttpClient#2383799::SendAsync() - An error occurred while sending HttpRequestMessage#21454193. System.Net.Http.HttpRequestException: An error occurred while sending the request. ---> System.Net.WebException: The underlying connection was closed: Could not establish trust relationship for the SSL/TLS secure channel. ---> System.Security.Authentication.AuthenticationException: The remote certificate is invalid according to the validation procedure.
at System.Net.TlsStream.EndWrite(IAsyncResult asyncResult)
at System.Net.PooledStream.EndWrite(IAsyncResult asyncResult)
at System.Net.ConnectStream.WriteHeadersCallback(IAsyncResult ar)
--- End of inner exception stack trace ---
at System.Net.HttpWebRequest.EndGetResponse(IAsyncResult asyncResult)
at System.Net.Http.HttpClientHandler.GetResponseCallback(IAsyncResult ar)
--- End of inner exception stack trace ---
System.Net Error: 0 : [2728] Exception in AppDomain#20234383::UnhandledExceptionHandler - One or more errors occurred..
at System.Threading.Tasks.Task.ThrowIfExceptional(Boolean includeTaskCanceledExceptions)
at System.Threading.Tasks.Task`1.GetResultCore(Boolean waitCompletionNotification)
at System.Threading.Tasks.Task`1.get_Result()
at ConsoleApp2.Program.Main(String[] args) in C:\Users\shane\source\repos\ConsoleApp2\ConsoleApp2\Program.cs:line 20
Looking at the log a bit more closely … we can see that the root certificate is not trusted, and therefore the HTTP request failed. Bingo!
System.Net Information: 0 : [8708] SecureChannel#42194754 - Remote certificate has errors:
System.Net Information: 0 : [8708] SecureChannel#42194754 - A certificate chain processed, but terminated in a root certificate which is not trusted by the trust provider.
System.Net Information: 0 : [8708] SecureChannel#42194754 - Remote certificate was verified as invalid by the user.
System.Net.Sockets Verbose: 0 : [8708] Socket#33711845::Dispose()
System.Net Error: 0 : [8708] Exception in HttpWebRequest#58870012:: - The underlying connection was closed: Could not establish trust relationship for the SSL/TLS secure channel..
System.Net Verbose: 0 : [8708] HttpWebRequest#58870012::EndGetResponse()
System.Net Error: 0 : [8708] Exception in HttpWebRequest#58870012::EndGetResponse - The underlying connection was closed: Could not establish trust relationship for the SSL/TLS secure channel..
System.Net.Http Error: 0 : [8708] Exception in HttpClientHandler#45004109::SendAsync - The underlying connection was closed: Could not establish trust relationship for the SSL/TLS secure channel..
at System.Net.HttpWebRequest.EndGetResponse(IAsyncResult asyncResult)
at System.Net.Http.HttpClientHandler.GetResponseCallback(IAsyncResult ar)
Final Thoughts
Although the above example is relatively rudimentary I hope you get the point and have learnt something new.
Although you may not need to use it. It’s good to keep this knowledge in your arsenal for when the time calls!
- Solved: Cmder – ‘ls’ is not recognized as an internal or external command, operable program or batch file. - 16th April 2024
- Solved: ‘Unable to find package specflow.plus.license’ error - 14th February 2024
- How to: Use GitHub Actions with OIDC to Authenticate with Azure - 16th November 2023
Leave a Reply